Hello world tutorial
Overview
In this tutorial, you will learn how to create a simple System Graph design that emulates a counter and displays the current counter value on a LCD screen emulated in Unity.
Create a System Graph Asset
Under the Asset folder, right-click and select Create > System > System Graph
Create the draft of the graph
Right-click and select Create a node script.
Set HelloWorld as the category name and Counter as the node name, then select Create.
After creating the script, you can now add it to a node. Right-click and select Create Node.
Type Counter in the search bar. You will also notice that your new node is located under the <category> group in your project folder.
Create a property and connect it to the node. Select the + button on the Property tab, then select the GameObject type. Enter the name LCDObject. Do not change any of its properties, as it connects to a node.
Drag the property onto the graph. It appears as an input port. Do not connect it.
In the system graph toolbar, select Save Asset.
Create scene objects
To create a Scene Object and link it to the node:
Drag the system graph into the scene.
The LCDObject requires the creation of a GameObject. The GameObject must then bind to the property. For this tutorial, the GameObject uses a Text object. Right-click on the
HelloWorld
object and select Select 3D Object > Text - TextMeshPro. Name the GameObject LCD.Bind the LCD GameObject to the Graph LCD Input property. Select the HelloWorld object,then drag the LCD object onto the
LCDObject
to bind it, or use the selection windows.
Code the nodes
Now that the graph is in the scene and connected to a GameObject through its input port, you can add a behaviour.
The Counter.cs
script describes a node that's in the HelloWorld system graph. This node has one input and one output, and connects to the graph input property.
When you open the Counter.cs
script, you'll find the following:
- A class
NodeCategory
header, which defines the node group, name, and tick mode. The node is Asynchronous by default. You must change the node to Synchronous for theOnTick
method to be called. - Two Field properties: one input and one output.
- An empty constructor.
- An empty
Enable
method. This method is called when the node, which links to other nodes at runtime, is ready to be initialized. - An empty
Disable
method. This method is called when the node is shutdown/disabled - An
OnTick
method. This method is called at a frequency defined in the SystemGraph Scheduler, when the node is Synchronous.
Open the Counter.cs
script in an IDE, and replace the existing content with the following:
using System;
using UnityEngine;
using Mechatronics.SystemGraph;
using TMPro;
[NodeCategory("HelloWorld", "Counter", NodeTick.Synchronous)]
public class Counter : NodeRuntime
{
[Field("LCD Display", PortDirection.Left, FieldExtra.Read), SerializeField]
private PortType<GameObject> lcdDisplayObject = new PortType<GameObject>();
[Field("Incrementation", PortDirection.Left, FieldExtra.Read), SerializeField]
private PortType<uint> incrementation = new PortType<uint>();
[Field("Output", PortDirection.Right, FieldExtra.Write), SerializeField]
private PortType<uint> output = new PortType<uint>();
private TextMeshPro _lcdDisplay;
Counter()
{
}
public override void Enable(Scheduler.ClockState clockState)
{
if (lcdDisplayObject != null && lcdDisplayObject.Read != null)
{
_lcdDisplay = lcdDisplayObject.Read.GetComponent<TextMeshPro>();
}
}
public override void Disable()
{
}
public override bool OnTick(double now, double eventTime, Scheduler.ClockState clockState, Scheduler.Signal signal)
{
output.Write = output.ReadRaw + incrementation.Read;
if (_lcdDisplay != null)
{
_lcdDisplay.text = String.Format("Count: {0}", output.Read.ToString());
}
return true;
}
}
This implements the Enable
and OnTick
methods behavior, and adds a new input port called Incrementation. This port should now display in the graph interface.
The Enable
method will retrieve the TextMeshPro
component and store its reference in the _lcdDisplay member.
The OnTick
method will increment the current output value by a step defined via the graph, or through another input. Once updated, it also changes the LCD display to reflect the new value. Since it uses the displayed value as a base for the incrementation, skipping this step would result in a constant output. There are other implementation methods, such as creating a closed loop.
Configure the graph
When you open the HelloWorld graph, the Incrementation input port should now be visible. Set the value in the edit field. If you connect an unsigned int
input to an int
type, you won't be able to use the edit field, as the port will be controlled by the input.
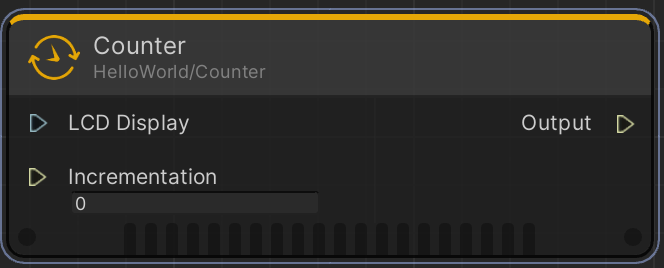
Note
As you set the increment manually, do not connect to any ports.
Connect the LCDObject to the LCD Display port and set a value in the Incrementation port edit field.
Set a clock frequency. This frequency calls the
OnTick
method. If you would like to create a seconds counter, set the frequency to1Hz
and theincrementation
value to1
.Double click the Counter Scheduler and set the frequency to
1Hz
. Do not change the prescaler value.Select Save Asset, exit the graph, and return to the Editor to test the system.
Test
In the Editor toolbar, select the Play button. The counter should now increment every second.